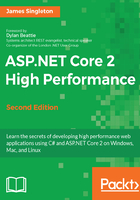
String interpolation
String interpolation is a more elegant and easier-to-work-with version of the familiar string format method. Instead of supplying the arguments to embed in the string placeholders separately, you can now embed them directly in the string. This is far more readable and less error-prone.
Let's demonstrate this with an example. Consider the following code that embeds an exception in a string:
catch (Exception e)
{
Console.WriteLine("Oh dear, oh dear! {0}", e);
}
This embeds the first (and in this case only) object in the string at the position marked by zero. It may seem simple, but it quickly gets complex if you have many objects and want to add another at the start. You then have to correctly renumber all the placeholders.
Instead, you can now prefix the string with a dollar character and embed the object directly in it. This is shown in the following code that behaves the same as the previous example:
catch (Exception e)
{
Console.WriteLine($"Oh dear, oh dear! {e}");
}
The ToString() method on an exception outputs all the required information, including the name, message, stack trace, and any inner exceptions. There is no need to deconstruct it manually; you may even miss things if you do.
You can also use the same format strings as you are used to. Consider the following code that formats a date in a custom manner:
Console.WriteLine($"Starting at: {DateTimeOffset.UtcNow:yyyy/MM/dd HH:mm:ss}");
When this feature was being built, the syntax was slightly different. So, be wary of any old blog posts or documentation that may not be correct.