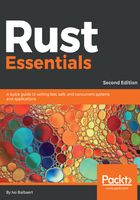
Printing with string interpolation
An obvious way to use variables is to print out their value, as is done here:
// see Chapter 2/code/constants2.rs static MAX_HEALTH: i32 = 100; static GAME_NAME: &str = "Monster Attack"; const MYPI: f32 = 3.14; fn main() { println!("The Game you are playing is called {}.", GAME_NAME); println!("You start with {} health points.", MAX_HEALTH); }
This gives an output which looks like this:
The Game you are playing is called Monster Attack.
You start with 100 health points.
The first argument of the println! macro is a literal format string containing a placeholder {}. The value of the constant or variable after the comma is converted to a string and comes in its place. There can be more than one placeholder and they can be numbered in order, so that they can be used repeatedly, as in the following code:
println!("In the Game {0} you start with {1} % health, yes you read it correctly: {1} points!", GAME_NAME, MAX_HEALTH);
This produces the following output:
In the Game Monster Attack you start with 100 % health, yes you read it correctly: 100 points!
The placeholder can also contain one or more named arguments, like this:
println!("You have {points} % health", points = 70);
This produces the following output:
You have 70 % health
Special ways of formatting can be indicated inside the {} after a colon (:), optionally prefixed by a position, like this:
println!("MAX_HEALTH is {:x} in hexadecimal", MAX_HEALTH); //
This gives an output like this: 64
println!("MAX_HEALTH is {:b} in binary", MAX_HEALTH); //
This gives an output like this: 1100100
println!( "Two written in binary is {0:b}", 2); //
This gives an output like this: 10
println!("pi is {:e} in floating point notation", PI); //
This gives an output like this: 3.14e0.
The following formatting possibilities exist:
- o: For octal
- x: For lower hexadecimal
- X: For upper hexadecimal
- p: For a pointer
- b: For binary
- e: For lower exponential notation
- E: For upper exponential notation
- ?: For debugging purposes
The format! macro has the same parameters and works the same way as the println! macro, but it returns a string instead of printing out.