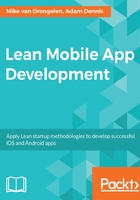
Data layer
Switching from one service provider to another (key partner) is easy if your app is well structured. Use a separate layer for accessing data and define contracts for the communication between your data layers and your controllers. Contracts are known as an interface (for Android) or as a protocol (for iOS). They contain no implementation and are nothing but appointments between one class and another. They define what methods are available, what parameters are required, and what the result type will be.
For example, let's say we are getting data from some kind of source. In the interface IRepository, we will define the names, results, and parameters for all methods that represent some operation. To be more precise, let's say we want to retrieve company news that we have stored somewhere in the cloud. It could be at Parse server (at Back4App or elsewhere), Amazon, Azure, or Firebase, it does not really matter where and how exactly we will get this data. Since it is an interface, we do not have to care about the actual implementation yet.
For Android, it could look like this:
public interface IRepository{ public void getNews(OnRepositoryResult handler, GetNewsRequest request);
For IOS, it looks like this (in Swift 2.x):
protocol RepositoryProtocol { func getNews(handler: RepositoryResultDelegate, request: GetNewsRequest)
The data layer classes that implement this interface or protocol will perform the actual job. They will retrieve the data from a remote data source.
For example, the Android implementation begins like this:
public class RemoteRepository implements IRepository { ... @Override public void getNews(OnRepositoryResult handler, GetNewsRequest request) { // Get data asynchronously and return the result }
While the IOS implementation begins like this:
public class RemoteRepository: RepositoryProtocol { ... func getNews(handler: RepositoryResultDelegate, request: GetNewsRequest){
In Chapter 8, Cloud Solutions for App Experiments, we will see what an implementation with Firebase will look like.
The data layer could also obtain the data from locally mocked or stubbed data. You can easily switch between the different sources. This makes it a great solution for testing purposes too.