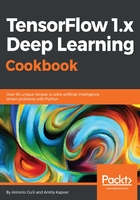
上QQ阅读APP看书,第一时间看更新
How to do it...
Here is how we proceed with the recipe:
- The first step is to import all the packages that we will need:
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
- We need to normalize the feature data since the data ranges of all the features are varied. We define a normalize function for it. Also, here we combine the bias to weights by adding another input always fixed to one value; to do this we define the function append_bias_reshape(). This technique is sometimes used to simplify programming:
def normalize(X)
""" Normalizes the array X """
mean = np.mean(X)
std = np.std(X)
X = (X - mean)/std
return X
def append_bias_reshape(features,labels):
m = features.shape[0]
n = features.shape[1]
x = np.reshape(np.c_[np.ones(m),features],[m,n + 1])
y = np.reshape(labels,[m,1])
return x, y
- Now we load the Boston house price dataset using TensorFlow contrib datasets and separate it into X_train and Y_train. Observe that this time, X_train contains all features. We can choose to normalize the data here, we also use append the bias and reshape the data for the network:
# Data
boston = tf.contrib.learn.datasets.load_dataset('boston')
X_train, Y_train = boston.data, boston.target
X_train = normalize(X_train)
X_train, Y_train = append_bias_reshape(X_train, Y_train)
m = len(X_train) #Number of training examples
n = 13 + 1 # Number of features + bias
- Declare the TensorFlow placeholders for the training data. Observe the change in the shape of the X placeholder.
# Placeholder for the Training Data
X = tf.placeholder(tf.float32, name='X', shape=[m,n])
Y = tf.placeholder(tf.float32, name='Y')
- We create TensorFlow variables for weight and bias. This time, weights are initialized with random numbers:
# Variables for coefficients
w = tf.Variable(tf.random_normal([n,1]))
- Define the linear regression model to be used for prediction. Now we need matrix multiplication to do the task:
# The Linear Regression Model
Y_hat = tf.matmul(X, w)
- For better differentiation, we define the loss function:
# Loss function
loss = tf.reduce_mean(tf.square(Y - Y_hat, name='loss'))
- Choose the right optimizer:
# Gradient Descent with learning rate of 0.01 to minimize loss
optimizer = tf.train.GradientDescentOptimizer(learning_rate=0.01).minimize(loss)
- Define the initialization operator:
# Initializing Variables
init_op = tf.global_variables_initializer()
total = []
- Start the computational graph:
with tf.Session() as sess:
# Initialize variables
sess.run(init_op)
writer = tf.summary.FileWriter('graphs', sess.graph)
# train the model for 100 epcohs
for i in range(100):
_, l = sess.run([optimizer, loss], feed_dict={X: X_train, Y: Y_train})
total.append(l)
print('Epoch {0}: Loss {1}'.format(i, l))
writer.close()
w_value, b_value = sess.run([w, b])
- Plot the loss function:
plt.plot(total)
plt.show()
Here too, we find that loss decreases as the training progresses: