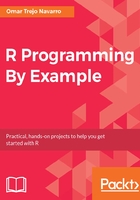
Characters
Text can be used just as easily, you just need to remember to use quotation marks (" ") around it. The following example shows how to save the text Hi, there! and "10" in two variables. Note that since "10.5" is surrounded by quotation marks, it is text and not a numeric value. To find what type of object you're actually dealing with you can use the class(), typeof(), and str() (short for structure) functions to get the metadata for the object in question.
In this case, since the y variable contains text, we can't multiply it by 2, as is seen in the error we get. Also, if you want to know the number of characters in a string, you can use the nchar() function, as follows:
x <- "Hi, there!" y <- "10" class(y)
#> [1] "character" typeof(y)
#> [1] "character" str(y)
#> chr "10" y * 2
#> Error in y * 2: non-numeric argument to binary operator nchar(x)
#> [1] 10 nchar(y)
#> [1] 2
Sometimes, you may have text information, as well as numeric information that you want to combine into a single string. In this case, you should use the paste() function. This function receives an arbitrary number of unnamed arguments, which is something we will define more precisely in a later section in this chapter. It then transforms each of these arguments into characters, and returns a single string with all of them combined. The following code shows such an example. Note how the numeric value of 10 in y was automatically transformed into a character type so that it could be pasted inside the rest of the string:
x <- "the x variable" y <- 10 paste("The result for", x, "is", y)
#> [1] "The result for the x variable is 10"
Other times, you will want to replace some characters within a piece of text. In that case, you should use the gsub() function, which stands for global substitution. This function receives the string to be replaced as its first argument, the string to replace with as its second argument, and it will return the text in the third argument with the corresponding replacements:
x <- "The ball is blue" gsub("blue", "red", x)
#> [1] "The ball is red"
Yet other times, you will want to know whether a string contains a substring, in which case you should use the gprel() function. The name for this function comes from terminal command known as grep, which is an acronym for global regular expression print (yes, you can also use regular expressions to look for matches). The l at the end of grepl() comes from the fact that the result is a logical:
x <- "The sky is blue" grepl("blue", x)
#> [1] TRUE grepl("red", x)
#> [1] FALSE