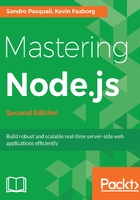
The Querystring module
As we saw with the URL module, query strings often need to be parsed into a map of key/value pairs. The Querystring module will either decompose an existing query string into its parts, or assemble a query string from a map of key/value pairs.
For example, querystring.parse("foo=bar&bingo=bango") will return:
{
foo: 'bar',
bingo: 'bango'
}
If our query strings are not formatted using the normal "&" separator and "=" assignment character, the Querystring module offers customizable parsing.
The second argument to Querystring can be a custom separator string, and the third, a custom assignment string. For example, the following will return the same mapping as given previously on a query string with custom formatting:
let qs = require("querystring");
console.log(qs.parse("foo:bar^bingo:bango", "^", ":"));
// { foo: 'bar', bingo: 'bango' }
You can compose a query string using the Querystring.stringify method:
console.log(qs.stringify({ foo: 'bar', bingo: 'bango' }));
// foo=bar&bingo=bango
As with parse, stringify also accepts custom separator and assignment arguments:
console.log(qs.stringify({ foo: 'bar', bingo: 'bango' }, "^", ":"));
// foo:bar^bingo:bango
Query strings are commonly associated with GET requests, seen following the ? character. As we saw previously, in these cases, automatic parsing of these strings using the url module is the most straightforward solution. However, strings formatted in such a manner also show up when we're handling POST data, and in these cases, the Querystring module is of real use. We'll discuss this usage shortly, but first, something about HTTP headers.