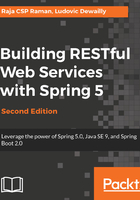
getUser – implementation in the handler and repository
Here, we will define and implement the getUser method in our repository. Also, we will call the getUser method in the main class through UserHandler.
We will add an abstract method for the getUser method in the UserRepository class:
Mono<User> getUser(Integer id);
Here, we will add the code for the getUser method. You can see that we have used the Mono return type for single-resource access.
In the UserRepositorySample class (the concrete class for UserRepository), we will implement the abstract method getUser:
@Override
public Mono<User> getUser(Integer id){
return Mono.justOrEmpty(this.users.get(id));
}
In the preceding code, we have retrieved the specific user by id. Also, we have mentioned that if the user is not available, the method should be asked to return an empty Mono.
In the UserHandler method, we will talk about how to handle the request and apply our business logic to get the response:
public Mono<ServerResponse> getUser(ServerRequest request){
int userId = Integer.valueOf(request.pathVariable("id"));
Mono<ServerResponse> notFound = ServerResponse.notFound().build();
Mono<User> userMono = this.userRepository.getUser(userId);
return userMono
.flatMap(user -> ServerResponse.ok().contentType(APPLICATION_JSON).body(fromObject(user)))
.switchIfEmpty(notFound);
}
In the preceding code, we have just converted the string id to an integer in order to supply it to our Repository method (getUser). Once we receive the result from the Repository, we are just mapping it in to Mono<ServerResponse> with the JSON content type. Also, we use switchIfEmpty to send the proper response if no item is available. If the searching item is not available, it will simply return the empty Mono object as a response.
Finally, we will add getUser in our routing path, which is in Server.java:
public RouterFunction<ServerResponse> routingFunction() {
UserRepository repository = new UserRepositorySample();
UserHandler handler = new UserHandler(repository);
return nest (
path("/user"),
nest(
accept(MediaType.ALL),
route(GET("/"), handler::getAllUsers)
)
.andRoute(GET("/{id}"), handler::getUser)
);
}
In the preceding code, we have just added a new entry, .andRoute(GET("/{id}"), handler::getUser), in our existing routing path. By doing so, we have added the getUser method and the corresponding REST API part to access a single user. After restarting the server, we should be able to use the REST API.