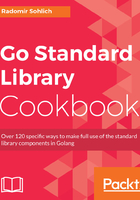
上QQ阅读APP看书,第一时间看更新
How to do it...
- Open the console and create the folder chapter02/recipe06.
- Navigate to the directory.
- Create the replace.go file with the following content:
package main
import (
"fmt"
"strings"
)
const refString = "Mary had a little lamb"
const refStringTwo = "lamb lamb lamb lamb"
func main() {
out := strings.Replace(refString, "lamb", "wolf", -1)
fmt.Println(out)
out = strings.Replace(refStringTwo, "lamb", "wolf", 2)
fmt.Println(out)
}
- Run the code by executing go run replace.go.
- See the output in the Terminal:

- Create the replacer.go file with the following content:
package main
import (
"fmt"
"strings"
)
const refString = "Mary had a little lamb"
func main() {
replacer := strings.NewReplacer("lamb", "wolf", "Mary", "Jack")
out := replacer.Replace(refString)
fmt.Println(out)
}
- Run the code by executing go run replacer.go.
- See the output in the Terminal:

- Create the regexp.go file with the following content:
package main
import (
"fmt"
"regexp"
)
const refString = "Mary had a little lamb"
func main() {
regex := regexp.MustCompile("l[a-z]+")
out := regex.ReplaceAllString(refString, "replacement")
fmt.Println(out)
}
- Run the code by executing go run regexp.go.
- See the output in the Terminal:
