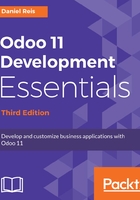
Extending Python methods
Python methods can also be extended for additional business logic. This is done by borrowing the mechanism Python already provides for inherited objects to extend their parent class behavior: super().
As an example, we will have the TodoTask class extend the ORM write() method to add some logic to it: when writing on a non-active record, it will automatically reactivate it.
Add this additional method in the models/todo_task_model.py file:
@api.multi def write(self, values): if 'active' not in values: values['active'] = True super().write(values)
The write() method expects an additional parameter, with a dictionary of values to write on the record. Unless a value is being explicitly set on the active field, we set it to True in case we are writing on an inactive record. We then use super() to call the parent write() method using the modified values.
super(TodoTask, self).write(values). This syntax also works with Python 3, so it should be preferred if keeping Python 2 compatibility is important.
We will have a chance to have a closer look at the ORM methods in Chapter 6, The ORM - Handling Application Data.