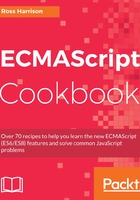
上QQ阅读APP看书,第一时间看更新
How to do it...
- Open your command-line application, navigate to your workspace, and create a new node package:
mkdir 02-creating-client-bundles cd 02-creating-client-bundles npm init -y
- Duplicate the main.js file from the Nesting modules under a single namespace recipe in Chapter 1, Building with Modules:
// main.js import { atlas, saturnV } from './rockets/index.js' export function main () { saturnV.launch(); atlas.launch(); }
- Create the rockets dependencies directory (these files can be copied from Nesting modules under a single namespace recipe in Chapter 1, Building with Modules):
// rockets/index.js import * as saturnV from './saturn-v.js'; import * as atlas from './atlas.js'; export { saturnV, atlas }; // rockets/launch-sequence.js export function launchSequence (countDownDuration, name) { let currCount = countDownDuration; console.log(`Launching in ${countDownDuration}`, name); const countDownInterval = setInterval(function () { currCount--; if (0 < currCount) { console.log(currCount); } else { console.log('%s LIFTOFF!!!', name); clearInterval(countDownInterval); } }, 1000); } // rockets/atlas.js import { launchSequence } from './launch-sequence.js'; const name = 'Atlas'; const COUNT_DOWN_DURATION = 20; export function launch () { launchSequence(COUNT_DOWN_DURATION, name); } // rockets/saturn-v.js import { launchSequence } from './launch-sequence.js'; export const name = "Saturn V"; export const COUNT_DOWN_DURATION = 10; export function launch () { launchSequence(COUNT_DOWN_DURATION, name); }
- Create an index.js file that loads and executes the main function from main.js:
// index.js import { main } from './main.js'; main();
- Install webpack:
> npm install --save-dev Webpack
- Create a webpack configuration file, named webpack.config.js, with an entry point at index.js and this output filename: bundle.js:
// webpack.config.js const path = require('path'); module.exports = { entry: './index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname) } };
- Add a build script to package.json:
{
/** package.json content**/
"scripts": {
"build": "webpack --config webpack.config.js"
}
}
- Run the webpack build to create bundle.js:
> npm run build
- You should see output that describes the build created and the modules contained therein. See the following output:
Hash: 5f2f1a7c077186c7a7a7 Version: webpack 3.6.0 Time: 134ms Asset Size Chunks Chunk Names bundle.js 6.7 kB 0 [emitted] main [0] ./rockets/launch-sequence.js 399 bytes {0} [built] [1] ./index.js 42 bytes {0} [built] [2] ./main.js 155 bytes {0} [built] [3] ./rockets/index.js 162 bytes {0} [built] [4] ./rockets/falcon-heavy.js 206 bytes {0} [built] [5] ./rockets/saturn-v.js 203 bytes {0} [built] [6] ./rockets/atlas.js 270 bytes {0} [built]
- Run the produced bundle.js file with node:
node ./bundle.js
- You should see the rockets count down and blast off.