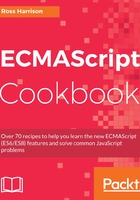
上QQ阅读APP看书,第一时间看更新
There's more...
It is possible to export named items directly. Consider the following file, atlas.js:
import { launchSequence } from './launch-sequence.js'; const name = 'Atlas'; const COUNT_DOWN_DURATION = 20; export const atlas = { name: name, COUNT_DOWN_DURATION: COUNT_DOWN_DURATION, launch: function () { launchSequence(COUNT_DOWN_DURATION, name); } };
The atlas member can be exported directly by index.js:
import * as falconHeavy from './falcon-heavy.js'; import * as saturnV from './saturn-v.js'; export { falconHeavy, saturnV }; export { atlas } from './atlas.js';
Then the main.js file can import the atlas member and launch it:
import { atlas, falconHeavy, saturnV } from './rockets/index.js' export function main () { saturnV.launch(); falconHeavy.launch(); atlas.launch(); }
This is one benefit of always using named exports; it's easier to collect and export specific members from packages with multiple modules.
Whether named or not, nesting is a great technique for grouping modules. It provides a mechanism for organizing code as the number of modules continues to grow.