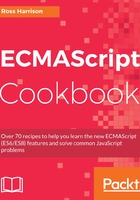
上QQ阅读APP看书,第一时间看更新
How to do it...
- Create a new folder with an index.html file, as seen in Exporting/importing multiple modules for external use.
- Inside of that directory, create a folder named rockets.
- Inside of rockets, create three files: falcon-heavy.js, saturn-v.js, and launch-sequence.js:
// falcon-heavy.js import { launchSequence } from './launch-sequence.js'; export const name = "Falcon Heavy"; export const COUNT_DOWN_DURATION = 5; export function launch () { launchSequence(COUNT_DOWN_DURATION, name); } (COUNT_DOWN_DURATION); } // saturn-v.js import { launchSequence } from './launch-sequence.js'; export const name = "Saturn V"; export const COUNT_DOWN_DURATION = 10; export function launch () { launchSequence(COUNT_DOWN_DURATION, name); } // launch-sequence.js export function launchSequence (countDownDuration, name) { let currCount = countDownDuration; console.log(`Launching in ${COUNT_DOWN_DURATION}`, name); const countDownInterval = setInterval(function () { currCount--; if (0 < currCount) { console.log(currCount); } else { console.log('%s LIFTOFF!!!', name); clearInterval(countDownInterval); } }, 1000); }
- Now create index.js, which exports the members of those files:
import * as falconHeavy from './falcon-heavy.js'; import * as saturnV from './saturn-v.js'; export { falconHeavy, saturnV };
- Create a main.js file (in the folder that contains rockets), which imports falconHeavey and saturnV from the index.js file and launches them:
import { falconHeavy, saturnV } from './rockets/index.js' export function main () { saturnV.launch(); falconHeavy.launch(); }
- Open in the browser, and see the following output:
