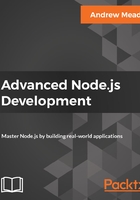
Connecting mongoose to database
The process of connecting is going to be pretty similar to what we did inside of our MongoDB scripts; for example, the mongodb-connect script. Here, we called MongoClient.connect, passing in a URL. What we're going to do for Mongoose is call mongoose.connect, passing in the exact same URL; mongodb is the protocol, call in //. We're going to be connecting to our localhost database on port 27017. Next up is going to be our /, followed by the database name, and we'll continue to use the TodoApp database, which we used over in the mongodb-connect script.
var mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/TodoApp');
This is where the two functions differ. The MongoClient.connect method takes a callback, and that is when we have access to the database. Mongoose is a lot more complex. This is good, because it means our code can be a lot simpler. Mongoose is maintaining the connection over time. Imagine I try to save something, save new something. Now obviously, by the time this save statement runs, mongoose.connect is not going to have had time to make a database request to connect. That's going to take a few milliseconds at least. This statement is going to run almost right away.
Behind the scenes, Mongoose is going to be waiting for the connection before it ever actually tries to make the query, and this is one of the great advantages of Mongoose. We don't have to micromanage the order in which things happen; Mongoose takes care of that for us.
There is one more thing I want to configure just above mongoose.connect. We've been using promises in this course, and we're going to continue using them. Mongoose supports callbacks by default, but callbacks really aren't how I like to program. I prefer promises as they're a lot simpler to chain, manage, and scale. Right above the mongoose.connect statement, we're going to tell Mongoose which promise library we want to use. If you're not familiar with the history of promises, it didn't have to always be something built into JavaScript. Promises originally came from libraries like Bluebird. It was an idea a developer had, and they created a library. People started using it, so much so that they added it to the language.
In our case, we need to tell Mongoose that we want to use the built- in promise library as opposed to some third-party one. We're going to set mongoose.Promise equal to global.Promise, and this is something we're only going to have to do once:
var mongoose = require('mongoose'); mongoose.Promise = global.Promise; mongoose.connect('mongodb://localhost:27017/TodoApp');
We're just going to put these two lines in server.js; we don't have to add them anywhere else. With this in place, Mongoose is now configured. We've connected to our database and we've set it up to use promises, which is exactly what we want. The next thing we're going to do is create a model.