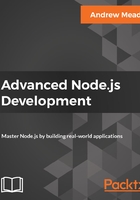
The deleteMany method
Now, we're going to start off with deleteMany, and we're going to target those duplicates we just created. The goal in this section, is to delete every single Todo inside of the Todos collection that has a text property equal to Eat lunch. Currently, there are three out of five that fit that criteria.
In Atom, we can go ahead and kick things off by doing db.collection. This is going to let us target our Todos collection. Now, we can go ahead and use the collection method deleteMany, passing in the arguments. In this case, the only argument we need is our object, and this object is just like the object we passed to find. With this, we can target our Todos. In this case, we're going to delete every Todo where the text equals Eat lunch.
//deleteMany db.collection('Todos').deleteMany({text: 'Eat lunch'});
We didn't use any punctuation in RoboMongo, so we're also going to avoid punctuation over in Atom; it needs to be exactly the same.
Now that we have this in place, we could go ahead and tack on a then call to do something when it either succeeds or fails. For now, we'll just add a success case. We are going to get a result argument passed back to the callback, and we can print that to the console.log(result) screen, and we'll take a look at exactly what is in this result object a bit later.
//deleteMany db.collection('Todos').deleteMany({text: 'Eat lunch'}).then((result) => { console.log(result); });
With this in place, we now have a script that deletes all Todos where the text value is Eat lunch. Let's go ahead and run it, and see exactly what happens. In the Terminal, I'm going to run this file. It's in the playground folder, and we just called it mongodb-delete.js:
node playground/mongodb-delete.js
Now when I run it, we get a lot of output:

A really important piece of output, the only important piece actually, is up at the very top. If you scroll to the top, what you're going to see is this result object. We get ok set to 1, which means things did go as expected, and we get n set to 3. n is the number of records that were deleted. In this case, we had three Todos that match that criteria, so three Todos were deleted. This is how you can target and delete many Todos.