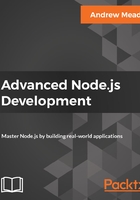
The find method
In Atom, what we're going to do is access the collection, just like we did inside of the mongodb-connect file using db.collection, passing in the collection name as the string. This collection is going to be the Todos collection. Now, we're going to go ahead and use a method available on collections called find. By default, we can call find with no arguments:
db.collection('Todos').find();
This means we're not providing a query, so we're not saying we want to fetch all Todos that are completed or not completed. We're just saying we want to fetch all Todos: everything, regardless of its values. Now, calling find is only the first step. find returns a MongoDB cursor, and this cursor is not the actual documents themselves. There could be a couple of thousand, and that would be really inefficient. It's actually a pointer to those documents, and the cursor has a ton of methods. We can use those methods to get our documents.
One of the most common cursor methods we're going to be using is .toArray. It does exactly what you think it does. Instead of having a cursor, we have an array of the documents. This means we have an array of objects. They have ID properties, text properties, and completed properties. This toArray method gets us exactly what we want back, which is the documents. toArray returns a promise. This means we can tack on a then call, we can add our callback, and when things go right, we can do something like print those documents to the screen.
db.collection('Todos').find().toArray().then((docs) => { });
We're going to get the documents as the first and only argument here, and we can also add an error handler. We'll get passed an error argument, and we can simply print something to the screen like console.log(Unable to fetch todos); as the second argument, we'll pass in the err object:
db.collection('Todos').find().toArray().then((docs) => { }, (err) => { console.log('Unable to fetch todos', err); });
Now, for the success case, what we're going to do is print the documents to the screen. I'm going to go ahead and use console.log to print a little message, Todos, and then I'll call console.log again. This time, we'll be using the JSON.stringify technique. I'll be passing in the documents, undefined for our filter function and 2 for our spacing.
db.collection('Todos').find().toArray().then((docs) => { console.log('Todos'); console.log(JSON.stringify(docs, undefined, 2)); }, (err) => { console.log('Unable to fetch todos', err); });
We now have a script that is capable of fetching the documents, converting them into an array, and printing them to the screen. Now, for the time being, I'm going to comment out the db.close method. Currently, that would interfere with our previous bit of code. Our final code would look as follows:
//const MongoClient = require('mongodb').MongoClient; const {MongoClient, ObjectID} = require('mongodb'); MongoClient.connect('mongodb://localhost:27017/TodoApp', (err, client) => { if(err){ console.log('Unable to connect to MongoDB server'); } console.log('Connected to MongoDB server'); const db = client.db('TodoApp');
db.collection('Todos').find().toArray().then((docs) => { console.log('Todos'); console.log(JSON.stringify(docs, undefined, 2)); }, (err) => { console.log('Unable to fetch todos', err); }); //client.close(); });
Save the file and run it from the Terminal. Inside of the Terminal, I'm going to go ahead and run our script. Obviously, since we connected to the database with Robomongo, it is running somewhere; it's running in this other tab. In the other tab, I can run the script. We're going to run it through node; it's in the playground folder, and the file itself is called mongodb-find.js:
node playground/mongodb-find.js
When I execute this file, we're going to get our results:

We have our Todos array with our two documents. We have our _id, our text properties, and our completed Boolean values. We now have a way to query our data right inside of Node.js. Now, this is a very basic query. We fetch everything in the Todos array, regardless of whether or not it has certain values.