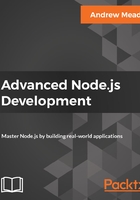
Error handling in mongodb-connect
Now, before we write any data, I'm going to go ahead and handle any potential errors that come about. I'll do that using an if statement. If there is an error, we're going to print a message to the console, letting whoever is looking at the logs know that we were unable to connect to the database server, console.log, then inside of quotes put something like Unable to connect to MongoDB server. After the if statement, we can go ahead and log out a success message, which will be something like console.log. Then, inside of quotes, we'll use Connected to MongoDB server:
MongoClient.connect('mongodb://localhost:27017/TodoApp', (err, client) => { if(err){ console.log('Unable to connect to MongoDB server'); } console.log('Connected to MongoDB server');
});
Now, when you're handling errors like this, the success code is going to run even if the error block runs. What we want to do instead is add a return statement right before the console.log('Unable to connect to MongoDB server'); line.
This return statement isn't doing anything fancy. All we're doing is using it to prevent the rest of the function from executing. As soon as you return from a function, the program stops, which means if an error does occur, the message will get logged, the function will stop, and we'll never see this Connected to MongoDB server message:
if(err) { return console.log('Unable to connect to MongoDB server'); }
An alternative to using the return keyword would be to add an else clause and put our success code in an else clause, but it's unnecessary. We can just use the return syntax, which I prefer.
Now, before we run this file, there is one more thing I want to do. At the very bottom of our callback function, we're going to call a method on db. It's called client.close:
MongoClient.connect('mongodb://localhost:27017/TodoApp', (err, client) => { if(err) { return console.log('Unable to connect to MongoDB server'); } console.log('Connected to MongoDB server');
const db = client.db('TodoApp');
client.close(); });
This closes the connection with the MongoDB server. Now that we have this in place, we can actually save the mongodb-connect file and run it inside of the Terminal. It doesn't do much yet, but it is indeed going to work.