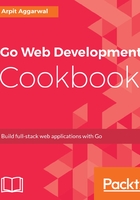
How it works…
Once we run the program, the HTTP server will start listening locally on port 8080.
Next, we will execute a couple of commands to see how the session works.
First, we will access /home by executing the following command:
$ curl -X GET http://localhost:8080/home
This will result in an unauthorized access message from the server as shown in the following screenshot:

This is because we first have to log in to an application, which will create a session ID that the server will validate before providing access to any web page. So, let's log in to the application:
$ curl -X GET -i http://localhost:8080/login
Executing the previous command will give us the Cookie, which has to be set as a request header to access any web page:

Next, we will use this provided Cookie to access /home, as follows:
$ curl --cookie "session-name=MTUyMzEwMTI3NXxEdi1CQkFFQ180SUFBUkFCRUFBQUpmLUNBQUVHYzNSeWFXNW5EQThBRFdGMWRHaGxiblJwWTJGMFpXUUVZbTl2YkFJQ0FBRT18ou7Zxn3qSbqHHiajubn23Eiv8a348AhPl8RN3uTRM4M=;" http://localhost:8080/home
This results in the home page as a response from the server:

Let's understand the Go program we have written:
- Using var store *sessions.CookieStore, we declared a private cookie store to store sessions using secure cookies.
- Using func init() { store = sessions.NewCookieStore([]byte("secret-key")) }, we defined an init() function that runs before main() to create a new cookie store and assign it to the store.
- Next, we defined a home handler where we get a session from the cookie store for the given name after adding it to the registry using store.Get and fetch the value of the authenticated key from the cache. If it is true, then we write Home Page to an HTTP response stream; otherwise, we write a You are unauthorized to view the page. message along with a 403 HTTP code.
- Next, we defined a login handler where we again get a session, set the authenticated key with a value of true, save it, and finally write You have successfully logged in. to an HTTP response stream.
- Next, we defined a logout handler where we get a session, set an authenticated key with the value of false, save it, and finally write You have successfully logged out. to an HTTP response stream.
- Finally, we defined main() where we mapped all handlers, home, login, and logout, to /home, /login, and /logout respectively, and start the HTTP server on localhost:8080.