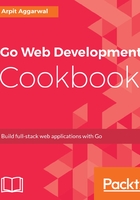
How it works…
Once we run the program, the HTTP server will start locally listening on port 8080. Browsing http://localhost:8080 will show us the File Upload Form, as shown in the following screenshot:

Pressing the Submit button after choosing a file will result in the creation of a file on the server with the name as uploadedFile inside the /tmp directory. You can see this by executing the following commands:

Also, the successful upload will display the message on the browser, as shown in the following screenshot:

Let's understand the Go program we have written:
We defined the fileHandler() handler, which gets the file from the request, reads its content, and eventually writes it onto a file on a server. As this handler does a lot of things, let’s go through it in detail:
- file, header, err := r.FormFile("file"): Here we call the FormFile handler on the HTTP request to get the file for the provided form key.
- if err != nil { log.Printf("error getting a file for the provided form key : ", err) return }: Here we check whether there is any problem while getting the file from the request. If there is, then log the error and exit with a status code of 1.
- defer file.Close(): The defer statement closes the file once we return from the function.
- out, pathError := os.Create("/tmp/uploadedFile"): Here we are creating a file named uploadedFile inside a /tmp directory with mode 666, which means the client can read and write but cannot execute the file.
- if pathError != nil { log.Printf("error creating a file for writing : ", pathError) return }: Here we check whether there are any problems with creating a file on the server. If there are, then log the error and exit with a status code of 1.
- _, copyFileError := io.Copy(out, file): Here we copy content from the file we received to the file we created inside the /tmp directory.
- fmt.Fprintf(w, "File uploaded successfully : "+header.Filename): Here we write a message along with a filename to an HTTP response stream.