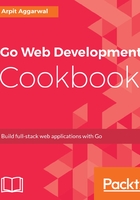
上QQ阅读APP看书,第一时间看更新
How to do it…
In this recipe, we will create a simple HTML form that has two input fields and a button to submit the form. Perform the following steps:
- Create login-form.html inside the templates directory, as follows:
$ mkdir templates && cd templates && touch login-form.html
- Copy the following content to login-form.html:
<html>
<head>
<title>First Form</title>
</head>
<body>
<h1>Login</h1>
<form method="post" action="/login">
<label for="username">Username</label>
<input type="text" id="username" name="username">
<label for="password">Password</label>
<input type="password" id="password" name="password">
<button type="submit">Login</button>
</form>
</body>
</html>
The preceding template has two textboxes—username and password—along with a Login button.
On clicking the Login button, the client will make a POST call to an action defined in an HTML form, which is /login in our case.
- Create html-form.go, where we will parse the form template and write it onto an HTTP response stream, as follows:
package main
import
(
"html/template"
"log"
"net/http"
)
const
(
CONN_HOST = "localhost"
CONN_PORT = "8080"
)
func login(w http.ResponseWriter, r *http.Request)
{
parsedTemplate, _ := template.ParseFiles("templates/
login-form.html")
parsedTemplate.Execute(w, nil)
}
func main()
{
http.HandleFunc("/", login)
err := http.ListenAndServe(CONN_HOST+":"+CONN_PORT, nil)
if err != nil
{
log.Fatal("error starting http server : ", err)
return
}
}
With everything in place, the directory structure should look like the following:

- Run the program with the following command:
$ go run html-form.go