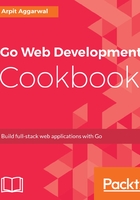
How it works…
Once we run the program, the HTTP server will start locally listening on port 8080 and accessing http://localhost:8080/, http://localhost:8080/login, and http://localhost:8080/logout from a browser or command line will render the message defined in the corresponding handler definition. For example, execute http://localhost:8080/ from the command line, as follows:
$ curl -X GET -i http://localhost:8080/
This will give us the following response from the server:

We could also execute http://localhost:8080/login from the command line as follows:
$ curl -X GET -i http://localhost:8080/login
This will give us the following response from the server:

Let's understand the program we have written:
- We started with defining three handlers or web resources, such as the following:
func helloWorld(w http.ResponseWriter, r *http.Request)
{
fmt.Fprintf(w, "Hello World!")
}
func login(w http.ResponseWriter, r *http.Request)
{
fmt.Fprintf(w, "Login Page!")
}
func logout(w http.ResponseWriter, r *http.Request)
{
fmt.Fprintf(w, "Logout Page!")
}
Here, the helloWorld handler writes Hello World! on an HTTP response stream. In a similar way, login and logout handlers write Login Page! and Logout Page! on an HTTP response stream.
- Next, we registered three URL paths—/, /login, and /logout with DefaultServeMux using http.HandleFunc() . If an incoming request URL pattern matches one of the registered paths, then the corresponding handler is called passing (http.ResponseWriter, *http.Request) as a parameter to it, as follows:
func main()
{
http.HandleFunc("/", helloWorld)
http.HandleFunc("/login", login)
http.HandleFunc("/logout", logout)
err := http.ListenAndServe(CONN_HOST+":"+CONN_PORT, nil)
if err != nil
{
log.Fatal("error starting http server : ", err)
return
}
}