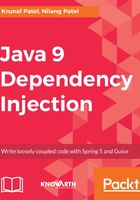
Inversion of object creation through the factory pattern
The factory pattern takes the responsibility of creating an object from a client who uses it. It generates the object of classes that are following a common interface. A client has to pass only type of the implementation it wants and the factory will create that object.
If we apply the factory pattern to our balance sheet example, the process of inverting of object creation is depicted as per the following diagram:

- Client (in our case, it's a balance sheet module) talks to the factory—Hey factory, can you please give me the fetch data object? Here is the type.
- The factory takes the type, creates the object, and passes it to the client (the balance sheet module).
- The factory can create the object of the same type only.
- The factory class is a complete black box for its clients. They know it's a static method to get objects.
The Balance Sheet module can get FetchData objects from FetchDataFactory. The code of FetchDataFactory will be as follows:
public class FetchDataFactory {
public static IFetchData getFetchData(String type){
IFetchData fetchData = null;
if("FROM_DB".equalsIgnoreCase(type)){
fetchData = new FetchDatabase();
}else if("FROM_WS".equalsIgnoreCase(type)){
fetchData = new FetchWebService();
}else {
return null;
}
return fetchData;
}
}
To use this factory, you need to update the configureFetchData() method of a balance sheet module as follows:
//Set the fetch data object from Factory.
public void configureFetchData(String type){
this.fetchDataObj = FetchDataFactory.getFetchData(type);
}
For export data, you need to create a separate factory as per the following snippet:
public class ExportDataFactory {
public static IExportData getExportData(String type){
IExportData exportData = null;
if("TO_HTML".equalsIgnoreCase(type)){
exportData = new ExportHTML();
}else if("TO_PDF".equalsIgnoreCase(type)){
exportData = new ExportPDF();
}else {
return null;
}
return exportData;
}
}
If a new fetch data or export data type is introduced, you need to change it in its respective factory class only.