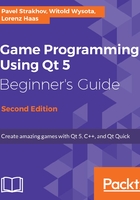
Graphics View architecture
The Graphics View Framework is part of the Qt Widgets module and provides a higher level of abstraction useful for custom 2D graphics. It uses software rendering by default, but it is very optimized and extremely convenient to use. Three components form the core of Graphics View, as shown:
- An instance of QGraphicsView, which is referred to as View
- An instance of QGraphicsScene, which is referred to as Scene
- Instances of QGraphicsItem, which are referred to as Items
The usual workflow is to first create a couple of items, add them to a scene, and then show that scene on a view:

After that, you can manipulate items from the code and add new items, while the user also has the ability to interact with visible items.
Think of the items as Post-it notes. You take a note and write a message on it, paint an image on it, both write and paint on it, or, quite possibly, just leave it blank. Qt provides a lot of item classes, all of which inherit QGraphicsItem. You can also create your own item classes. Each class must provide an implementation of the paint() function, which performs painting of the current item, and the boundingRect() function, which must return the boundary of the area the paint() function paints on.
What is the scene, then? Well, think of it as a larger sheet of paper on to which you attach your smaller Post-its, that is, the notes. On the scene, you can freely move the items around while applying funny transformations to them. It is the scene's responsibility to correctly display the items' position and any transformations applied to them. The scene further informs the items about any events that affect them.
Last, but not least, let's turn our attention to the view. Think of the view as an inspection window or a person who holds the paper with the notes in their hands. You can see the paper as a whole, or you can only look at specific parts. Also, as a person can rotate and shear the paper with their hands, so the view can rotate and shear the scene's content and do a lot more transformations with it. QGraphicsView is a widget, so you can use the view like any other widget and place it into layouts for creating neat graphical user interfaces.