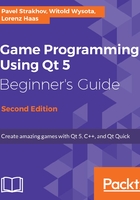
Protecting against invalid input
The configuration dialog did not have any validation until now. Let's make it such that the button to accept the dialog is only enabled when neither of the two line edits is empty (that is, when both the fields contain player names). To do this, we need to connect the textChanged signal of each line edit to a slot that will perform the task.
First, go to the configurationdialog.h file and create a private slot void updateOKButtonState(); in the ConfigurationDialog class (you will need to add the private slots section manually). Use the following code to implement this slot:
void ConfigurationDialog::updateOKButtonState() { QPushButton *okButton = ui->buttonBox->button(QDialogButtonBox::Ok); okButton->setEnabled(!ui->player1Name->text().isEmpty() && !ui->player2Name->text().isEmpty()); }
This code asks the button box that currently contains the OK and Cancel buttons to give a pointer to the button that accepts the dialog (we have to do that because the buttons are not contained in the form directly, so there are no fields for them in ui). Then, we set the button's enabled property based on whether both player names contain valid values or not.
Next, edit the constructor of the dialog to connect two signals to our new slot. The button state also needs to be updated when we first create the dialog, so add an invocation of updateOKButtonState() to the constructor:
ui->setupUi(this);
connect(ui->player1Name, &QLineEdit::textChanged,
this, &ConfigurationDialog::updateOKButtonState);
connect(ui->player2Name, &QLineEdit::textChanged,
this, &ConfigurationDialog::updateOKButtonState);
updateOKButtonState();