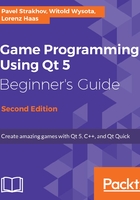
What just happened?
QWidget (and, by extension, any widget class) has a font property that determines the properties of the font this widget uses. This property has the QFont type. We can't just write label->font()->setBold(isBold);, because font() returns a const reference, so we have to make a copy of the QFont object. That copy has no connection to the label, so we need to call label->setFont(f) to apply our changes. To avoid repetition of this procedure, we created a helper function, called setLabelBold.
The last thing that needs to be done is to handle the situation when the game ends. Connect the gameOver() signal from the board to a new slot in the main window class. Implement the slot as follows:
void MainWindow::handleGameOver(TicTacToeWidget::Player winner) { QString message; if(winner == TicTacToeWidget::Player::Draw) { message = tr("Game ended with a draw."); } else { QString winnerName = winner == TicTacToeWidget::Player::Player1 ? ui->player1Name->text() : ui->player2Name->text(); message = tr("%1 wins").arg(winnerName); } QMessageBox::information(this, tr("Info"), message); }
This code checks who won the game, assembles the message (we will learn more about QString in Chapter 6, Qt Core Essentials), and shows it using a static method QMessageBox::information() that shows a modal dialog containing the message and a button that allows us to close the dialog.
Run the game and check that it now highlights the current player and shows the message when the game ends.