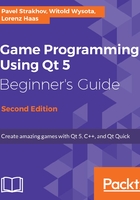
上QQ阅读APP看书,第一时间看更新
Time for action – Changing the texts on the labels from the code
Printing text to the console is not as impressive as changing the text in our form. We don't have GUI for letting users enter their names yet, so we'll hardcode some names for now. Let's change the implementation of our slot to the following:
void MainWindow::startNewGame() { ui->player1Name->setText(tr("Alice")); ui->player2Name->setText(tr("Bob")); }
Now, when you run the application and click on the button, the labels in the form will change. Let's break down this code into pieces:
- As mentioned earlier, the first label's object is accessible in our class as ui->player1Name and has the QLabel * type.
- We're calling the setText method of the QLabel class. This is the setter of the text property of QLabel (the same property that we edited in the property editor of the Design mode). As per Qt's naming convention, getters should have the same name as the property itself, and setters should have a set prefix, followed by the property name. You can set the text cursor on setText and press F1 to learn more about the property and its access functions.
- The tr() function (which is short for "translate") is used to translate the text to the current UI language of the application. We will describe the translation infrastructure of Qt in Chapter 6, Qt Core Essentials. By default, this function returns the passed string unchanged, but it's a good habit to wrap any and all string literals that are displayed to the user in this function. Any user-visible text that you enter in the form editor is also subject to translation and is passed through a similar function automatically. Only strings that should not be affected by translation (for example, object names that are used as identifiers) should be created without the tr() function.