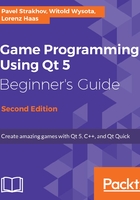
Time for action – Receiving the button-click signal from the form
Open the mainwindow.h file and create a private slots section in the class declaration, then declare the startNewGame() private slot, as shown in the following code:
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = nullptr);
~MainWindow();
private slots:
void startNewGame();
}
To quickly implement a freshly declared method, we can ask Qt Creator to create the skeleton code for us by positioning the text cursor at the method declaration, pressing Alt + Enter on the keyboard, and choosing Add definition in tictactoewidget.cpp from the popup.
Write the highlighted code in the implementation of this method:
void MainWindow::startNewGame() { qDebug() << "button clicked!"; }
Add #include <QDebug> to the top section of the mainwindow.cpp file to make the qDebug() macro available.
Finally, add a connect statement to the constructor after the setupUi() call:
ui->setupUi(this);
connect(ui->startNewGame, &QPushButton::clicked,
this, &MainWindow::startNewGame);
Run the application and try clicking on the button. The button clicked! text should appear in the Application Output pane in the bottom part of Qt Creator's window (if the pane isn't activated, use the Application Output button in the bottom panel to open it):
