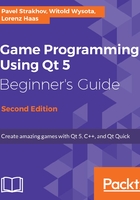
Old connect syntax
Before Qt 5, the old connect syntax was the only option. It looks as follows:
connect(spinBox, SIGNAL(valueChanged(int)), dial, SLOT(setValue(int)));
This statement establishes a connection between the signal of the spinBox object called valueChanged that carries an int parameter and a setValue slot in the dial object that accepts an int parameter. It is forbidden to put argument names or values in a
connect statement. Qt Creator is usually able to suggest all possible inputs in this context if you press Ctrl + Space after SIGNAL( or SLOT(.
While this syntax is still available, we discourage its wide use, because it has the following drawbacks:
- If the signal or the slot is incorrectly referenced (for example, its name or argument types are incorrect) or if argument types of the signals and the slot are not compatible, there will be no compile-time error, only a runtime warning. The new syntax approach performs all the necessary checks at compile time.
- The old syntax doesn't support casting argument values to another type (for example, connect a signal carrying a double value with a slot taking an int parameter).
- The old syntax doesn't support connecting a signal to a standalone function, a lambda expression, or a functor.
The old syntax also uses macros and may look unclear to developers not familiar with Qt. It's hard to say which syntax is easier to read (the old syntax displays argument types, while the new syntax displays the class name instead). However, the new syntax has a big disadvantage when using overloaded signals or slots. The only way to resolve the overloaded function type is to use an explicit cast:
connect(spinBox, static_cast<void (QSpinBox::*)(int)>(&QSpinBox::valueChanged),
...);
The old connect syntax includes argument types, so it doesn't have this issue. In this case, the old syntax may look more acceptable, but compile-time checks may still be considered more valuable than shorter code. In this book, we prefer the new syntax, but use the old syntax when working with overloaded methods for the sake of clarity.