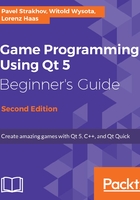
Creating signals and slots
If you create a QObject subclass (or a QWidget subclass, as QWidget inherits QObject), you can mark a method of this class as a signal or a slot. If the parent class had any signals or non-private slots, your class will also inherit them.
In order for signals and slots to work properly, the class declaration must contain the Q_OBJECT macro in a private section of its definition (Qt Creator has generated it for us). When the project is built, a special tool called Meta-Object Compiler (moc) will examine the class's header and generate some extra code necessary for signals and slots to work properly.
A signal can be created by declaring a class method in the signals section of the class declaration:
signals: void valueChanged(int newValue);
However, we don't implement such a method; this will be done automatically by moc. We can send (emit) the signal by calling the method. There is a convention that a signal call should be preceded by the emit macro. This macro has no effect (it's actually a blank macro), but it helps us clarify our intent to emit the signal:
void MyClass::setValue(int newValue) { m_value = newValue; emit valueChanged(newValue); }
You should only emit signals from within the class methods, as if it were a protected function.
Slots are class methods declared in the private slots, protected slots, or public slots section of the class declaration. Contrary to signals, slots need to be implemented. Qt will call the slot when a signal connected to it is emitted. The visibility of the slot (private, protected, or public) should be chosen using the same principles as for normal methods.
macro expands to public, and slots is a blank macro. So, the compiler treats them as normal methods. These keywords are, however, used by moc to determine how to generate the extra code.