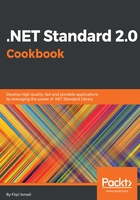
上QQ阅读APP看书,第一时间看更新
How to do it...
- Open Visual Studio 2017.
- Now, open the solution from the previous recipe. Click File | Open | Open Project/Solution, or press Ctrl + Shift + O, and select the Chapter2.Linq solution.
- Press Ctrl + Shift + B for a quick build to check that everything is fine.
- Now, click on the Chapter2.Linq solution label. Click File | Add | New Project.
- In the Add New Project template dialog box, expand the Visual C# node in the left-hand pane.
- Select Web and then ASP.NET Web Application (.NET Framework) in the right-hand pane:

- Now, in the Name: text box, type Chapter2.Linq.QueriesMVC as the name and leave the Location: text box at its default value:

- In the New ASP.NET Web Application dialog box, select Empty from the template list.
- Select MVC as the Add folders and core references for: option:

- Leave the rest as it is and click OK to create the default ASP.NET MVC Web Application template.
- Now, Solution Explorer should look like this:

- Now, right-click on the Controllers folder inside the Chapter2.Linq.QueriesMVC project.
- Select Add | Controller.
- In the Add Scaffold dialog box, select MVC 5 Controller - Empty:

- Click Add.
- In the Add Controller dialog box, type HomeController as the name of the controller:

- Click Add.
- Now, the Solution Explorer (press Ctrl + Alt + L) should look like this:

- Now, right-click on the References label and select Add Reference.
- In the Reference Manager, select Projects and check Chapter2.Linq.QueriesLib from the list:

- Click OK.
- Now, double-click on HomeController.cs in the Controllers folder.
- In the code window for HomeController.cs, scroll up and add the following code at the last line of the using directives:
using Chapter2.Linq.QueriesLib;
- Inside the Index() action, before the return keyword, add the following code:
var telephoneBook = new TelephoneBook();
ViewBag.Contacts = telephoneBook.GetContacts();
- Right-click on the method name of the Index() method and select Add View:

- In the Add View dialog box, leave the defaults and click Add:

- You will be presented with an Index.cshtml code window with the default template.
- Change the code <h2>Index</h2> as follows:
<h2>Contacts</h2>
- Now move the cursor to the bottom of the code window and add this code:
<ul>
@foreach(var contact in ViewBag.Contacts as List<string>)
{
<li>@contact</li>
}
</ul>
- Let's press F5 and test the code.
- By default, the browser will load http://localhost:portnumber/Home/ and here is the output:

- Now let's close the browser and switch back to the HomeController.cs code window.
- Right after the end curly bracket of the Index() action method, add the following code:
public ActionResult Search(string ln)
{
var telephoneBook = new TelephoneBook();
if (string.IsNullOrEmpty(ln))
{
ViewBag.Contacts = telephoneBook.GetContacts();
}
else
{
ViewBag.Contacts =
telephoneBook.GetContactsByLastName(ln);
}
return View();
}
- Right-click on the method name of the Search() action and select Add View.
- Follow steps 26 and 27 to add the Search.chtml view.
- In the Search.chtml code window, change the code <h2>Search</h2> to the following :
<h2>Search Results - Contacts</h2>
- Add the following code to Search.chtml after the <h2> tags:
<ul>
@foreach (var contact in ViewBag.Contacts as List<string>)
{
<li>@contact</li>
}
</ul>
- Let's press F5 and debug the current code.
- Type http://localhost:portnumber/Home/Search in the browser address bar and press Enter:

- Now again, type http://localhost:portnumber/Home/Search?ln=Marrier in the address bar and press Enter:

- Close the browser and switch back to the HomeController.cs code window.
- Add the following code after the Search() action method:
public ActionResult SortedContacts(bool asc = true)
{
var telephoneBook = new TelephoneBook();
ViewBag.Contacts = telephoneBook.GetSortedContacts(asc);
return View();
}
- Right-click on the method name of the SortedContacts() action and select Add View.
- Follow steps 26 and 27 to add the SortedContacts.chtml view.
- Now, in the SortedContacts.chtml, change <h2>SortedContacts</h2> to <h2>Sorted Contacts</h2>.
- At the end of the <h2> tags add the following code.
<ul>
@foreach (var contact in ViewBag.Contacts as List<string>)
{
<li>@contact</li>
}
</ul>
- Press F5 to debug the current code.
- Type http://localhost:portnumber/Home/SortedContacts in the address bar of the browser and press Enter:

- Again, type http://localhost:51084/Home/SortedContacts?asc=false in the address bar and press Enter:

- Close the browser.