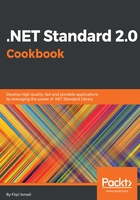
上QQ阅读APP看书,第一时间看更新
How to do it...
- Open Visual Studio 2017.
- Click File | New | Project and, in the New Project template dialog box, select Visual Studio Solutions under the Other Project Types node in the left-hand pane and then Blank Solution in the right-hand pane.
- In the Name: text box, type Chapter2.Linq as the name of the solution. Select a preferred location under the Location: drop-down list or click the Browse... button and select a location. Leave the defaults as they are:
- Click OK.
- Now, in the Solution Explorer (or press Ctrl + Alt + L), select Chapter2.Linq. Right-click and select Add | New Project.
- In the Add New Project dialog box, expand the Visual C# node and select .NET Standard in the left-hand pane.
- In the right-hand pane, select Class Library (.NET Standard).

- Now, in the Name: text box, type Chapter2.Linq.QueriesLib and leave the Location: text box as it is:

- Click OK.
- Now, the Solution Explorer (press Ctrl + Alt + L to open) should look like this:

- Select Class1.cs in the project tree and press F2.
- Rename Class1.cs as TelephoneBook.cs, also making sure that you have done the same to the class name itself.
- Answer Yes in the confirmation dialog box for renaming.
- Now, the Solution Explorer should look like this:

- Double-click the TelephoneBook.cs file to open the code window.
- Now, scroll up to the top of the code window and add the following code next to the last line of the using directives:
using System.Collections.Generic;
using System.Linq;
- Again, scroll down till you reach the open curly bracket of the TelephoneBook class and add the following code:
private List<string> _contactList;
- Now, in the next line, add the following code as the constructor method:
public TelephoneBook()
{
_contactList = new List<string>();
_contactList.Add("Lenna Paprocki");
_contactList.Add("Donette Foller");
_contactList.Add("Simona Morasca");
_contactList.Add("Mitsue Tollner");
_contactList.Add("Leota Dilliard");
_contactList.Add("Sage Wieser");
_contactList.Add("Kris Marrier");
_contactList.Add("Minna Amigon");
_contactList.Add("Abel Maclead");
_contactList.Add("Kiley Caldarera");
_contactList.Add("Graciela Ruta");
}
- Next to the constructor, add the following code:
public List<string> GetContacts()
{
return _contactList;
}
- Again, add this code block at the end of the GetContacts() method:
public List<string> GetContactsByLastName(string lastName)
{
var contacts = _contactList.Where(
c => c.Contains(lastName)).ToList();
return contacts;
}
- Finally, add the following code block at the end of the GetContactsByLastName() method:
public List<string> GetSortedContacts(bool ascending = true)
{
var sorted = _contactList.OrderBy(c => c).ToList();
if (!ascending)
{
sorted = _contactList.OrderByDescending(c => c).ToList();
}
return sorted;
}
- Now that we are done with adding code to the .NET Standard 2.0 library, let's hit Ctrl + Shift + B for a quick build and check for syntax errors.