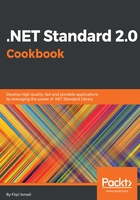
How it works...
Let's have a look at the steps and try to understand. In steps 1 to 3, we opened an existing solution that contained the .NET Standard 2.0 library. We did a quick build to check that all the syntax was OK and the project is compiling without any issues. In steps 4 to 8, we added a .NET Core console-based application to the project and gave it a proper name.
In steps 10 to 14, we added a reference to the .NET Standard 2.0 library project from our .NET Core console application. In step 17, we added two namespaces to the Program.cs code. One is for accessing reflection classes, which are contained in the System.Reflection namespace. The other one is the .NET Standard 2.0 library itself.
In step 18, we added code to the Program.cs, Main() method:
MemberInfo info = typeof(Calculator);
Console.WriteLine($"Assembly Name: {info.Name}");
Console.WriteLine($"Module Name: {info.Module.Name}");
Console.WriteLine();
In the first line of code we have stored a Calculator class type into a variable named info, which is a type of System.Reflections.MemberInfo(). In the second line of code, we have accessed the of the assembly class name using a Name property, while again we have accessed the module name using a Module.Name property. The Member Info() class allows you to get information about the attributes of a member and provides access to member metadata:
var calculator = new Calculator();
var typeObject = calculator.GetType();
var methods = typeObject.GetRuntimeMethods();
foreach (var method in methods)
{
Console.WriteLine($"Method name : {method.Name},
---> Return type : {method.ReturnType}");
}
Console.ReadLine();
In the preceding few lines of code, we have created an instance of the Calculator() class established inside the .NET Standard 2.0 library. In the second line, we stored the type of class. In the third line, we have a variable named methods to store all the available runtime methods inside that class. Since GetRuntimeMethods() is a collection, we can easily use a foreach statement to iterate through the collections. And finally, inside the foreach() statement we have output the name and the return type of each method available inside the Calculator() class.
At the end, we waited for the user to press any key to exit the console application.
In step 19, in the output, you might have noticed that apart from the two public methods we have created, there are several more methods. These are coming from the base Object class itself.