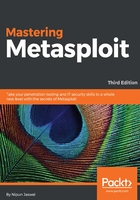
Regular expressions
Regular expressions are used to match a string or its number of occurrences in a given set of strings or a sentence. The concept of regular expressions is critical when it comes to Metasploit. We use regular expressions in most cases while writing fuzzers, scanners, analyzing the response from a given port, and so on.
Let's have a look at an example of a program that demonstrates the usage of regular expressions.
Consider a scenario where we have a variable, n, with the value Hello world, and we need to design regular expressions for it. Let's have a look at the following code snippet:
irb(main):001:0> n = "Hello world" => "Hello world" irb(main):004:0> r = /world/ => /world/ irb(main):005:0> r.match n => #<MatchData "world"> irb(main):006:0> n =~ r => 6
We have created another variable called r and stored our regular expression in it, namely, /world/. In the next line, we match the regular expression with the string using the match object of the MatchData class. The shell responds with a message, MatchData "world", which denotes a successful match. Next, we will use another approach of matching a string using the =~ operator, which returns the exact location of the match. Let's see one other example of doing this:
irb(main):007:0> r = /^world/ => /^world/ irb(main):008:0> n =~ r => nil irb(main):009:0> r = /^Hello/ => /^Hello/ irb(main):010:0> n =~ r => 0 irb(main):014:0> r= /world$/ => /world$/ irb(main):015:0> n=~ r => 6
Let's assign a new value to r, namely, /^world/; here, the ^ operator tells the interpreter to match the string from the start. We get nil as an output if it is not matched. We modify this expression to start with the word Hello; this time, it gives us back the location 0, which denotes a match as it starts from the very beginning. Next, we modify our regular expression to /world$/, which denotes that we need to match the word world from the end so that a successful match is made.
For further information on regular expressions in Ruby, refer to: http://www.tutorialspoint.com/ruby/ruby_regular_expressions.htm.
Refer to a quick cheat sheet for using Ruby programming efficiently at the following links: https://github.com/savini/cheatsheets/raw/master/ruby/RubyCheat.pdf and http://hyperpolyglot.org/scripting.
Refer to http://rubular.com/ for more on building correct regular expressions.