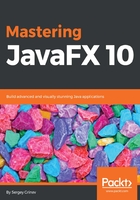
上QQ阅读APP看书,第一时间看更新
Button and Event Handlers
The first and most common control is button's family. Button has an EventHandler that is called when Button is clicked (or fired). All code in the event handler is always run on JavaFX Application Thread:
// chapter2/other/ButtonDemo.java
Button btn = new Button();
btn.setText("Say 'Hello World'");
btn.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
System.out.println("Hello World!");
}
});
In addition to text, you can use any Node inside a button:
Button btn = new Button();
btn.setText("Say 'Hello World'");
btn.setGraphic(new Circle(10));
Button can be assigned the roles of default button or cancel button, responding to Enter or Esc correspondingly:
btn.setDefaultButton(true);
//or
btn.setCancelButton(true);
Button can be easily assigned with a mnemonic (Alt + letter) by using the _ sign:
btn.setText("_Press Alt+P to fire me."):

Note you can disable this behavior by calling btn.setMnemonicParsing(false);.
There are also 4 more classes that share a common ancestor (ButtonBase) and most functionality with Button:
- ToggleButton saves state clicked/unclicked and changes visuals accordingly. Its state can be retrieved by calling the isSelected() method.
- CheckBox has the same behavior as ToggleButton but different visuals and API. CheckBox state is controlled by the getState() method.
- Hyperlink is a button with no extra decorations, which also remembers if it was already clicked.
- MenuButton is a part of the Menu API that allows you to create user and context menus.