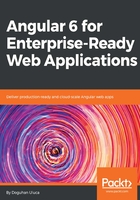
Implementing an HTTP GET operation
Now, we can implement the GET call in the Weather service:
- Add a new function to the WeatherService class named getCurrentWeather
- Import the environment object
- Implement the httpClient.get function
- Return the results of the HTTP call:
src/app/weather/weather.service.ts
import { environment } from '../../environments/environment'
...
export class WeatherService {
constructor(private httpClient: HttpClient) { }
getCurrentWeather(city: string, country: string) {
return this.httpClient.get<ICurrentWeatherData>(
`${environment.baseUrl}api.openweathermap.org/data/2.5/weather?` +
`q=${city},${country}&appid=${environment.appId}`
)
}
}
Note the use of ES2015's String Interpolation feature. Instead of building your string by appending variables to one another like environment.baseUrl + 'api.openweathermap.org/data/2.5/weather?q=' + city + ',' + country + '&appid=' + environment.appId, you can use the backtick syntax to wrap `your string`. Inside the backticks, you can have newlines and also directly embed variables into the flow of your string by wrapping them with the ${dollarbracket} syntax. However, when you introduce a newline in your code, it will be interpreted as a literal newline—\n. In order to break up the string in your code, you may add a backslash \, but then the next line of your code can have no indentation. It is easier to just concatenate multiple templates, as shown in the preceding code sample.
Note the use TypeScript Generics with the get function using the caret syntax like <TypeName>. Using generics is development-time quality of life feature. By providing the type information to the function, input and/or return variables types of that function will be displayed as your write your code and validated during development and also at compile time.