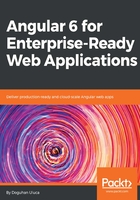
Coding style
You can customize the coding style enforcement and code generation behavior in VS Code and Angular CLI. When it comes to JavaScript, I prefer StandardJS settings, which codifies a minimal approach to writing code, while maintaining great readability. This means 2-spaces for tabs and no semicolons. In addition to the reduced keystrokes, StandardJS also takes less space horizontally, which is especially valuable when your IDE can only utilize half of the screen with the other half taken up by the browser. You can read more about StandardJS at: https://standardjs.com/.
With the default settings, your code will look like this:
import { AppComponent } from "./app.component";
With StandardJS settings, your code will look like this:
import { AppComponent } from './app.component'
Ultimately, this is an optional step for you. However, my code samples will follow the StandardJS style. You can start making the configuration changes by following these steps:
- Install the Prettier - Code formatter extension
- Update .vscode/extensions.json file with the new extension
- Execute npm i -D prettier
You can use i for install and -D instead of the more verbose --save-dev option. However, if you mistype -D as -d, you will end up saving the package as a production dependency.
- Edit package.json with a new script, update the existing ones, and create new formatting rules:
package.json
...
"scripts": {
...
"standardize": "prettier **/*.ts --write",
"start": "npm run standardize && ng serve --port 5000",
"build": "npm run standardize && ng build",
...
},
...
"prettier": {
"printWidth": 90,
"semi": false,
"singleQuote": true,
"trailingComma": "es5",
"parser": "typescript"
}
...
macOS and Linux users must modify the standardize script to add single-quotes around **/*.ts for the script to correctly traverse the directory. In macOS and Linux, the correct script looks like "standardize": "prettier '**/*.ts' --write".
- Similarly, update tslint.json with new formatting rules:
tslint.json ...
"quotemark": [ true, "single" ], ... "semicolon": [ true, "never" ],
...
"max-line-length": [
true,
120
],
...
- Execute npm run standardize to update all your files to the new style
- Observe all the file changes in GitHub Desktop
- Going forward, every time you execute npm start or npm run build, the new standardize script will automatically run and keep the formatting of your files in shape
- Commit and push your changes to your repository
As you type in new code or generate new components using Angular CLI, you will encounter double-quotes or semicolons being underlined with a red-squiggly line to indicate an issue. In most of those cases, a yellow bulb icon will appear next to the issue. If you click on the bulb, you will see an action to Fix: Unnecessary semicolon or a similar message. You can either take advantage of these auto-fixers or press Shift + Alt + F to run the Prettier Format Document command on the entire file. In the following screenshot, you can see the auto-fixer in action with the yellow bulb and the corresponding contextual menu:

VS Code Auto-Fixer