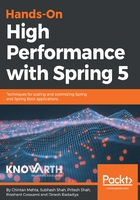
Reduction in implementing code
All application classes are simple POJO classes; Spring is not invasive. It does not require you to extend framework classes or implement framework interfaces for most use cases. Spring applications do not require a Jakarta EE application server, but they can be deployed on one.
Before the Spring Framework, typical J2EE applications contained a lot of plumbing code. For example:
- Code for getting a database connection
- Code for handling exceptions
- Transaction management code
- Logging code and a lot more
Let's look at the following simple example of executing a query using PreparedStatement:
PreparedStatement st = null;
try {
st = conn.prepareStatement(INSERT_ACCOUNT_QUERY);
st.setString(1, bean.getAccountName());
st.setInt(2, bean.getAccountNumber());
st.execute();
}
catch (SQLException e) {
logger.error("Failed : " + INSERT_ACCOUNT_QUERY, e);
} finally {
if (st != null) {
try {
st.close();
} catch (SQLException e) {
logger.log(Level.SEVERE, INSERT_ACCOUNT_QUERY, e);
}
}
}
In the preceding example, there are four lines of business logic and more than 10 lines of plumbing code. The same logic can be applied in a couple of lines using the Spring Framework, as follows:
jdbcTemplate.update(INSERT_ACCOUNT_QUERY,
bean.getAccountName(), bean.getAccountNumber());
Using Spring, you can use a Java method as a request handler method or remote method, like a service() method of a servlet API, but without dealing with the servlet API of the servlet container. It supports both XML-based and annotation-based configuration.
Spring enables you to use a local Java method as a message handler method, without using a Java Message Service (JMS) API in the application. Spring serves as a container for your application objects. Your objects do not have to worry about finding and establishing connections with each other. Spring also enables you to use the local Java method as a management operation, without using a Java Management Extensions (JMX) API in the application.