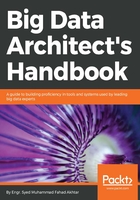
上QQ阅读APP看书,第一时间看更新
Driver program
The driver program will utilize the MapReduce framework in the Hadoop environment to calculate the total amount paid for each bill. It is the primary executioner as it contains the main method. It defines all of the parameters required by the Hadoop framework to process a program. Following is the complete code for our driver class; note that comments have been added to make the code self-explanatory:
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class UtilityBillSummary {
public static void main(String[] args) throws Exception {
//Initiate Hadoop Configuration class
Configuration conf = new Configuration();
// It is to configure a MapReduce Job and assigning it a name
// for logging purposes
Job job = Job.getInstance(conf, "UtilityBillSummary");
job.setJarByClass(UtilityBillSummary.class);
// In this step we will define what are the names of out Mapper
// and Reducer classes
job.setMapperClass(UtilityMapper.class);
job.setReducerClass(UtilityReducer.class);
// Here we are defining the types of <Key, Value> pairs
// Key will be of Text type i.e. String
// Value will be of Long type
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(LongWritable.class);
// Here we are assigning the input and output files
// which will be passed while executing program
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
// Wait until MapReduce Job finishes
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}