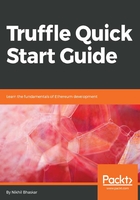
上QQ阅读APP看书,第一时间看更新
Wrapping up
Your final TaskMaster.sol file should look like this:
pragma solidity ^0.4.17;
contract TaskMaster {
mapping (address => uint) public balances; // balances of everyone
address public owner; // owner of the contract
function TaskMaster() public {
owner = msg.sender;
balances[msg.sender] = 10000;
}
function reward(address doer, uint rewardAmount)
public
isOwner()
hasSufficientFunds(rewardAmount)
returns(bool sufficientFunds)
{
balances[msg.sender] -= rewardAmount;
balances[doer] += rewardAmount;
return sufficientFunds;
}
function getBalance(address addr) public view returns(uint) {
return balances[addr];
}
modifier isOwner() {
require(msg.sender == owner);
_;
}
modifier hasSufficientFunds(uint rewardAmount) {
require(balances[msg.sender] >= rewardAmount);
_;
}
}
You can also find this file in the repo, under contracts (https://github.com/PacktPublishing/Truffle-Quick-Start-Guide/blob/master/chapter1/contracts/TaskMaster.sol).
Now that we've created a full TaskMaster.sol file, you can do the following bit of housekeeping.
Delete all contents of migrations/2_deploy_contracts.js and replace them with the following code:
var TaskMaster = artifacts.require("./TaskMaster.sol");
module.exports = function(deployer) {
deployer.deploy(TaskMaster);
};
This specifies that TaskMaster.sol should be deployed to a blockchain. You will learn more about migrations in Chapter 4, Migrating Your Dapp to Ethereum Blockchains.