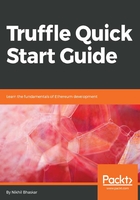
上QQ阅读APP看书,第一时间看更新
Securing your contract with modifiers
To ensure that our contract is secure, we ensure the following:
- Only an owner can call the reward function
- The owner of the contract has sufficient funds to transfer to the doer
Let's add a few modifiers, isOwner and hasSufficientFunds:
pragma solidity ^0.4.17;
contract TaskMaster {
mapping (address => uint) public balances; // balances of everyone
address public owner; // owner of the contract
function TaskMaster() public {
owner = msg.sender;
balances[msg.sender] = 10000;
}
function reward(address doer, uint rewardAmount)
public
isOwner()
hasSufficientFunds(rewardAmount)
returns(bool sufficientFunds)
{
balances[msg.sender] -= rewardAmount;
balances[doer] += rewardAmount;
return sufficientFunds;
}
modifier isOwner() {
require(msg.sender == owner);
_;
}
modifier hasSufficientFunds(uint rewardAmount) {
require(balances[msg.sender] >= rewardAmount);
_;
}
}
isOwner requires that the sender of the reward function is the owner of the contract.
hasSufficientFunds requires that the sender of the contract has enough funds to reward the doer.