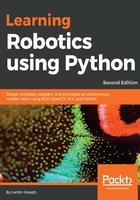
上QQ阅读APP看书,第一时间看更新
Hello_world_subscriber.py
The subscriber code is as follows:
#!/usr/bin/env python import rospy from std_msgs.msg import String
The following code is a callback function that is executed when a message reaches the hello_pub topic. The data variable contains the message from the topic, and it will print using rospy.loginfo():
def callback(data): rospy.loginfo(rospy.get_caller_id()+"I heard %s",data.data)
The following steps will start the node with a hello_world_subscriber name and start subscribing to the /hello_pub topic:
- The data type of the message is String, and when a message arrives on this topic, a method called callback will be called:
def listener(): rospy.init_node('hello_world_subscriber',
anonymous=True) rospy.Subscriber("hello_pub", String, callback)
- The following code will keep your node from exiting until the node is shut down:
rospy.spin()
- The following is the main section of the Python code. The main section will call the listener() method, which will subscribe to the /hello_pub topic:
if __name__ == '__main__': listener()
- After saving two Python nodes, you need to change the permission to executable using the chmod commands:
chmod +x hello_world_publisher.py chmod +x hello_world_subscriber.py
- After changing the file permission, build the package using the catkin_make command:
cd ~/catkin_ws catkin_make
- The following command adds the current ROS workspace path in all terminals so that we can access the ROS packages inside this workspace:
echo "source ~/catkin_ws/devel/setup.bash" >> ~/.bashrc source ~/.bashrc
The following is the output of the subscriber and publisher nodes:

Output of the hello world node
- First, we need to run roscore before starting the nodes. The roscore command or ROS master is needed to communicate between nodes. So, the first command is as follows:
$ roscore
- After executing roscore, run each node using the following commands:
-
- The following command will run the publisher:
$ rosrun hello_world hello_world_publisher.py
-
- The following command will run the subscriber node. This node subscribes to the hello_pub topic, as shown in the following code:
$ rosrun hello_world hello_world_subscriber.py
We have covered some of the basics of ROS. Now, we will see what Gazebo is and how we can work with Gazebo using ROS.