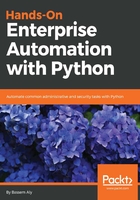
Using the telnet protocol in Python
Telnet is one of the oldest protocols available in the TCP/IP stack. It is used primarily to exchange data over an established connection between a server and client. It uses TCP port 23 in the server for listening to the incoming connection from the client.
In our case, we will create a Python script that acts as a telnet client, and other routers and switches in the topology will act as the telnet server. Python comes with a native support for telnet via a library called telnetlib so we don't need to install it.
After creating the client object by instantiating it from the Telnet() class, available from the telnetlib module, we can use the two important functions available inside telnetlib, which are read_until() (used to read the output) and write() (used to write on the remote device). Both functions are used to interact with the created channel, either by writing or reading the output returned from it.
Also, it's important to note that reading the channel using read_until() will clear the buffer and data won't be available for any further reading. So, if you read important data and you will process and work on it later, then you need to save it as a variable before you continue with your script.
Telnet data is sent in clear text format, so your credentials and password may be captured and viewed by anyone performing a man-in-the-middle attack. Some service providers and enterprises still use it and integrate it with VPNs and radius/tacacs protocols to provide lightweight and secure access.
Follow the steps to understand the whole script:
- We will import the telnetlib module inside our Python script and define the username and passwords in variables, as in the following code snippet:
import telnetlib
username = "admin"
password = "access123"
enable_password = "access123"
- We will define a variable that establishes the connection with the remote host. Note that we won't provide the username or password during connection establishment; we will only provide the IP address of the remote host:
cnx = telnetlib.Telnet(host="10.10.88.110") #here we're telnet to Gateway
- Now we will provide the username for the telnet connection by reading the returned output from the channel and searching for the Username: keyword. Then we write our admin username. The same process is used when we need to enter the telnet password and enable password:
cnx.read_until("Username:")
cnx.write(username + "\n")
cnx.read_until("Password:")
cnx.write(password + "\n")
cnx.read_until(">")
cnx.write("en" + "\n")
cnx.read_until("Password:")
cnx.write(enable_password + "\n")
It's important to provide the exact keywords that appear in the console when you establish the telnet connection or the connection, will enter an infinite loop. Then Python script will be timed out with an error.
- Finally, we will write the show ip interface brief command on the channel and read till the router prompt # to get the output. This should get us the interface configuration in the router:
cnx.read_until("#")
cnx.write("show ip int b" + "\n")
output = cnx.read_until("#")
print output
The full script is:

The script output is:

Notice that the output contains the executed command show ip int b, and the router prompt "R1#" is returned and printed in the stdout. We could use built-in string functions like replace() to clean them from the output:
cleaned_output = output.replace("show ip int b","").replace("R1#","")
print cleaned_output

As you noticed, we provided both the password and enable password as clear text inside our script, which is considered a security issue. It's also not good practice to hardcode the values inside your Python script. Later, in the next section, we will hide the password and design a mechanism to provide credentials during script runtime only.
Also, if you want to execute commands that span multiple pages in output like show running config then you will need to disable paging first by sending terminal length 0 after connecting to the device and before sending the command to it.