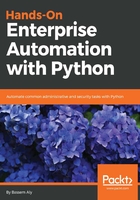
Configuring devices using netmiko
Netmiko can be used to configure remote devices over SSH. It does that by accessing config mode using the .config method and then applies the configuration given in list format. The list itself can be provided inside the Python script or read from the file, then converted to a list using the readlines() method:
from netmiko import ConnectHandler
SW2 = {
'device_type': 'cisco_ios',
'ip': '10.10.88.112',
'username': 'admin',
'password': 'access123',
'secret': 'access123',
}
core_sw_config = ["int range gig0/1 - 2","switchport trunk encapsulation dot1q",
"switchport mode trunk","switchport trunk allowed vlan 1,2"]
print "########## Connecting to Device {0} ############".format(SW2['ip'])
net_connect = ConnectHandler(**SW2)
net_connect.enable()
print "***** Sending Configuration to Device *****"
net_connect.send_config_set(core_sw_config)
In the previous script, we did the same thing that we did before to connect to SW2 and enter enable mode, but this time we leveraged another netmiko method called send_config_set(), which takes the configuration in list format and accesses device configuration mode and starts to apply it. We have a simple configuration that modifies the gig0/1 and gig0/2 and applies trunk configuration on them. You can check if the command executed successfully by running show run command on the device; you should get output similar to the following:
