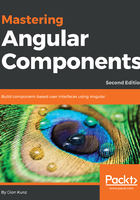
Main application component
Let's take a look at our main application component. You can think of it as the outermost component of your application. It's called the main component because it represents your whole application. This is where your component tree has its roots and therefore it's sometimes also called the root component.
First, let's look at the component TypeScript file located in src/app/app.component.ts:
import {Component} from '@angular/core';
@Component({
selector: 'mac-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'mac';
}
There's nothing different here from what we already know about structuring a component, something that we learned in the previous chapter. However, there are two main differences here compared to how we created the components before. Instead of using the template property to write our HTML template inline, we're using the templateUrl property to tell Angular to load the HTML from the specified file. The second thing which we've not covered yet is how to load CSS for your component. The styleUrls property allows us to specify an array of URLs which get resolved to assemble the styles of our component. Similar to the HTML template mechanism, we could also use a property called styles to write our styles inline within the component TypeScript file.
For our application, we'd like to change the behaviour slightly on how we handle styling. The default way of organizing styles when creating components is that each component contains its own encapsulated styles. However, for our project, we want to use the global styles.css file to add all our component styles. This will make it much easier to work with the books source repository and eliminates the need to include CSS code excerpts within this book.
By default, Angular uses a Shadow DOM emulation on our components, which is preventing styles within a component to leak outside and influence other components. However, this behaviour can be changed easily by configuring the view encapsulation on components.
Angular has three ways to handle view encapsulation and each way has its own pros and cons. Let's look at the different settings:

Let's change the view encapsulation of our main component to use the ViewEncapsulation.None mode. Since we're going to put all our styles in the global src/styles.css file, we can also remove the styleUrls property from our component configuration completely:
import {Component, ViewEncapsulation} from '@angular/core';
@Component({
selector: 'mac-root',
templateUrl: './app.component.html',
encapsulation: ViewEncapsulation.None
})
export class AppComponent {
title = 'mac';
}