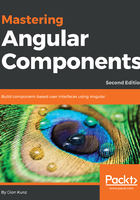
Encapsulation
Encapsulation is a very important factor when thinking about maintenance in a system. Having a classical OOP background, I've learned that encapsulation means bundling logic and data together into an isolated container. This way, we can operate on the container from the outside and treat it like a closed system.
There are many positive aspects of this approach when it comes to maintainability and accessibility. Dealing with closed systems is important for the organization of our code. However, this is even more important because we can organize ourselves while working with code:

I have a pretty bad memory, and it's very important for me to find the right focus level when working on code. Immediate memory research has tells us that the human brain can remember about seven items at once on average. Therefore, it's crucial for us to write code in such a way that it allows us to focus on fewer and smaller pieces at once.
A clear encapsulation helps us in organizing our code. We can perhaps forget all the internals of the closed system and about the kind of logic and data that we've put into it. We should focus only on its surface, which allows us to work on a higher-abstraction level. Similar to the previous diagram, without using a hierarchy of encapsulated components, we'd have all our code cobbled together on the same level.
Encapsulation encourages us to isolate small and concise components and build a system of components. During development, we can focus on the internals of one component and only deal with the interface of other components.
Sometimes, we forget that all the organization of the coding we actually perform is for ourselves and not for the computer that runs this code. If this was for the computer, then we would probably all start writing in machine language again. A strong encapsulation helps us access specific code easily, focus on one layer of the code, and trust the underlying implementations within capsules.
The following TypeScript example shows you how to use encapsulation to write maintainable applications. Let's assume that we are in a T-shirt factory, and we need some code to produce T-shirts with a background and foreground color. This example uses some language features of TypeScript. If you're not familiar with the language features of TypeScript, don't worry too much at this point. We will learn about these later in this chapter:
// This class implements data and logic to represent a color
// which establishes clean encapsulation.
class Color {
constructor(private red: number, private green: number, private blue: number) {}
// Using this function we can convert the internal color values
// to a hex color string like #ff0000 (red).
getHex(): string {
return '#' + Color.getHexValue(this.red) + Color.getHexValue(this.green) +
Color.getHexValue(this.blue);
}
// Static function on Color class to convert a number from
// 0 to 255 to a hexadecimal representation 00 to ff
static getHexValue(number): string {
const hex = number.toString(16);
return hex.length === 2 ? hex : '0' + hex;
}
}
// Our TShirt class expects two colors to be passed during
// construction that will be used to render some HTML
class TShirt {
constructor(private backgroundColor: Color, private foregroundColor: Color) {}
// Function that returns some markup which represents our T-Shirts
getHtml(): string {
return `
<t-shirt style="background-color: ${this.backgroundColor.getHex()}">
<t-shirt-text style="color: ${this.foregroundColor.getHex()}">
Awesome Shirt!
</t-shirt-text>
</t-shirt>
`;
}
}
// Instantiate a blue colour
const blue: Color = new Color(0, 0, 255);
// Instantiate a red color
const red: Color = new Color(255, 0, 0);
// Create a new shirt using the above colours
const awesomeShirt: TShirt = new TShirt(blue, red);
// Adding the generated markup of our shirt to our document
document.body.innerHTML = awesomeShirt.getHtml();
Using a clean encapsulation, we can now work with the abstraction of color in our T-shirt. We don't need to worry about how to calculate the hexadecimal representation of colors at the T-shirt level because this is already done by the Color class. This makes your application maintainable and keeps it very open for change.
I strongly recommend that you read about the SOLID principles if you haven't done so already. As the name already suggests, this assembly of principles is a solid power tool that can change the way you organize code tremendously. You can learn more about the SOLID principles in the book Agile Principles, Patterns, and Practices, by Robert C. Martin.