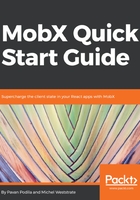
上QQ阅读APP看书,第一时间看更新
when() with a promise
There is a special version of when(), which takes only one argument (the predicate function), and gives back a promise instead of the disposer function. This is a nice trick where you can skip using the effect function and instead wait for when() to resolve before executing the effect. This is easier to see in code, as shown here:
class Inventory {
/* ... */
async trackAvailability(name) {
// 1. Wait for availability
await when(() => {
const item = this.items.find(x => x.name === name);
return item ? item.quantity > 0 : false;
});
// 2. Execute side-effect
console.log(`${name} is now available`);
}
/* ... */
}
In comment 1, we are waiting for the availability of the item using when() that only takes the predicate function. By using the async-await operators to wait for the promise, we get clean, readable code. Any code that follows the await statement is automatically scheduled to execute after the promise resolves. This is a nicer form of using when() if you prefer not to pass an effect callback.
when() is also very efficient and does not poll the predicate function to check for changes. Instead, it relies on the MobX reactivity system to re-evaluate the predicate function, when the underlying observables change.