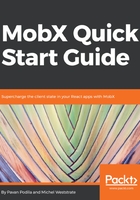
Observing the state changes
Observables alone cannot make an interesting system. We also need their counterparts, the observers. MobX gives you three different kinds of observers, each tailor-made for the use cases you will encounter in your application. The core observers are autorun, reaction, and when. We will look at each of them in more detail in the next chapter, but let's introduce autorun for now.
The autorun API takes a function as its input and executes it immediately. It also keeps track of the observables that are used inside the passed-in function. When these tracked observables change, the function is re-executed. What is really beautiful and elegant about this simple setup is that there is no extra work required to track observables and subscribe to any changes. It all just happens automatically. It's not magic, per se, but definitely an intelligent system at work, which we will cover in a later section:
import {observable, autorun} from 'mobx';
let cart = observable({
itemCount: 0,
modified: new Date()
});
autorun(() => {
console.log(`The Cart contains ${cart.itemCount} item(s).`);
});
cart.itemCount++;
// Console output:
The Cart contains 0 item(s).
The Cart contains 1 item(s).
In the preceding example, the arrow-function that was passed into autorun is executed for the first time and also when itemCount is incremented. This results in two console logs being printed. autorun makes the passed-in function (the tracking-function) an observer of the observables it references. In our case, cart.itemCount was being observed and when it was incremented, the tracking function was automatically notified, resulting in the console logs getting printed.