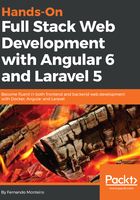
The component life cycle
In the life cycle of an Angular component, after instantiating, the component runs a definite path of execution from its beginning until its end. The most basic way to understand this is through observing the following code:
export class HelloComponent implements OnInit, OnDestroy {
constructor() { }
ngOnInit() {
... Some code goes here
}
ngOnDestroy() {
... Some code goes here
}
}
In the preceding example, you can see methods called ngOnInit() and ngOnDestroy; the names are very intuitive, and show us that we have a beginning and an end. The ngOnInit() method is implemented through its OnInit interface, and the same goes for the ngOnDestroy() method. As you saw in the previous chapter, the interfaces in TypeScript are very useful – it's not any different here.
In the following diagram, we will look at the main interfaces that we can implement on a component. In the diagram, after the Constructor() method, there are eight interfaces (also known as hooks); each one is responsible for one thing, at a specific moment:

We will not describe the interfaces one by one in this chapter, so as not to overload you, but, throughout the course of the book, we will use them in the application that we will build. Also, the preceding link includes detailed information about each interface and hook.