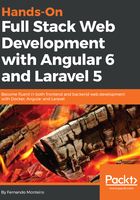
上QQ阅读APP看书,第一时间看更新
Creating a class
The best way to understand classes in TypeScript is to create one. A simple class looks like the following code:
class Band {
public name: string;
constructor(text: string) {
this.name = text;
}
}
Let's create our first class:
- Open your text editor and create a new file, called my-first-class.ts, and add the following code:
class MyBand {
// Properties without prefix are public
// Available is; Private, Protected
albums: Array<string>;
members: number;
constructor(albums_list: Array<string>, total_members: number) {
this.albums = albums_list;
this.members = total_members;
}
// Methods
listAlbums(): void {
console.log("My favorite albums: ");
for(var i = 0; i < this.albums.length; i++) {
console.log(this.albums[i]);
}
}
}
// My Favorite band and his best albums
let myFavoriteAlbums = new MyBand(["Ace of Spades", "Rock and Roll", "March or Die"], 3);
// Call the listAlbums method.
console.log(myFavoriteAlbums.listAlbums());
We added some comments to the previous code to facilitate understanding.
A class can have as many methods as necessary. In the case of the previous class, we gave only one method, to list our favorite band albums. You can test this piece of code on your Terminal, passing any information that you want inside the new MyBand() constructor.
This is pretty simple, and if you've had some contact with Java, C#, or even PHP, you will have already seen this class structure.
Here, we can apply the inheritance (OOP) principle to our class. Let's see how to do it:
- Open the band-class.ts file and add the following code, right after the console.log() function:
/////////// using inheritance with TypeScript ////////////
class MySinger extends MyBand {
// All Properties from MyBand Class are available inherited here
// So we define a new constructor.
constructor(albums_list: Array<string>, total_members: number) {
// Call the parent's constructor using super keyword.
super(albums_list, total_members);
}
listAlbums(): void{
console.log("Singer best albums:");
for(var i = 0; i < this.albums.length; i++) {
console.log(this.albums[i]);
}
}
}
// Create a new instance of the YourBand class.
let singerFavoriteAlbum = new MySinger(["At Falson Prision", "Among out the Stars", "Heroes"], 1);
console.log(singerFavoriteAlbum.listAlbums());
In Angular, classes are very useful for defining components, as we will see in the Chapter 3, Understand the core concepts of Angular 6.