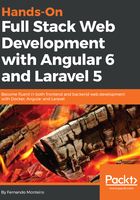
Using the resource flag to create CRUD methods
Let's see another feature of the Artisan CLI, creating all of the Create, Read, Update, and Delete (CRUD) operations using a single command.
First, in the app/Http/Controllers folder, delete the BandController.php file:
- Open your Terminal window and type the following command:
php artisan make:controller BandController --resource
This action will create the same file again, but now, it includes the CRUD operations, as shown in the following code:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class BandController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
//
}
/**
* Show the form for creating a new resource.
*
* @return \Illuminate\Http\Response
*/
public function create()
{
//
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
//
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
//
}
/**
* Show the form for editing the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function edit($id)
{
//
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
//
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
//
}
}
For this example, we will write only two methods: one to list all of the records, and another to get a specific record. Don't worry about the other methods; we will cover all of the methods in the upcoming chapters.
- Let's edit public function index() and add the following code:
$bands = Band::all();
return $bands;
- Now, edit public function show() and add the following code:
$band = Band::find($id);
return view('bands.show', array('band' => $band));
- Add the following line, right after App\Http\Requests:
use App\Band;
- Update the routes.php file, inside the routes folder, to the following code:
Route::get('/', function () {
return view('welcome');
});
Route::resource('bands', 'BandController');
- Open your browser and go to http://localhost:8081/bands, where you will see the following content:
[{
"id": 1,
"name": "porro",
"description": "Minus sapiente ut libero explicabo et voluptas harum.",
"created_at": "2018-03-02 19:20:58",
"updated_at": "2018-03-02 19:20:58"}
...]
Don't worry if your data is different from the previous code; this is due to Faker generating random data. Note that we are returning a JSON directly to the browser, instead of returning the data to the view. This is a very important feature of Laravel; it serializes and deserializes data, by default.