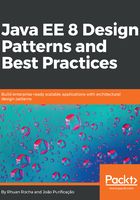
Implementing the Transaction class
The Transaction class is responsible for controlling the transaction lifecycle and defining the transaction delimit:
package com.packt.javaee8.domainstore;
import javax.annotation.PostConstruct;
public class Transaction {
private boolean opened;
@PostConstruct
public void init(){
this.opened = false;
}
public void commit() throws Exception {
if( !opened ) throw new Exception("Transaction is not opened");
opened = false;
}
public void rollback() throws Exception {
if( !opened ) throw new Exception("Transaction is not opened");
opened = false;
}
public void begin() throws Exception {
if( opened ) throw new Exception("Transaction already is opened");
opened = true;
}
public boolean isOpened(){
return opened;
}
}
In the preceding code block, we have the Transaction class, which is responsible for controlling the transaction lifecycle and defining the transaction delimit. This class has the init() method, annotated with @PostConstruct, which configures this method to be called after the constructor is executed. Furthermore, this class has the commit method, which is used when the user needs to confirm the transaction; the rollback method, used to undo all transactions; the begin method, used to open a transaction; and the isOpened method, which is used to verify whether a transaction is open or not.
The transaction is closed if the begin method was not called or if the commit or rollback methods were called and a new call to the begin method was not made.